Internet of Things
4. Building an IoT cloud application
Lesson 1: Setup Arduino Development Environment
Setup Arduino Development Environment - Internet of Things - ثاني ثانوي
Part 1
1. IoT Fundamentals
2. The IoT in Our Lives
3. Building IoT applications with Arduino
4. Building an IoT cloud application
Part 2
5. IoT Advanced Applications
6. ++IoT Programming With C
7. IoT messaging
8. IoT Wireless Sensor Network Simulation
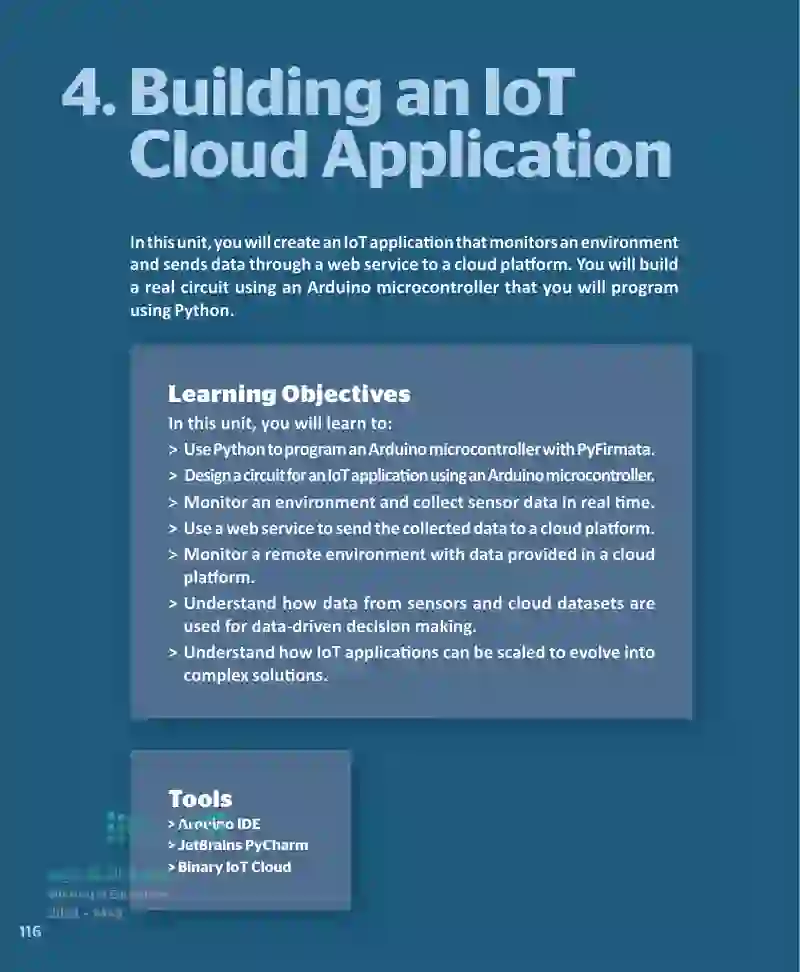
In this unit, you will create an IoT application that monitors an environment
Learning Objectives
Tools
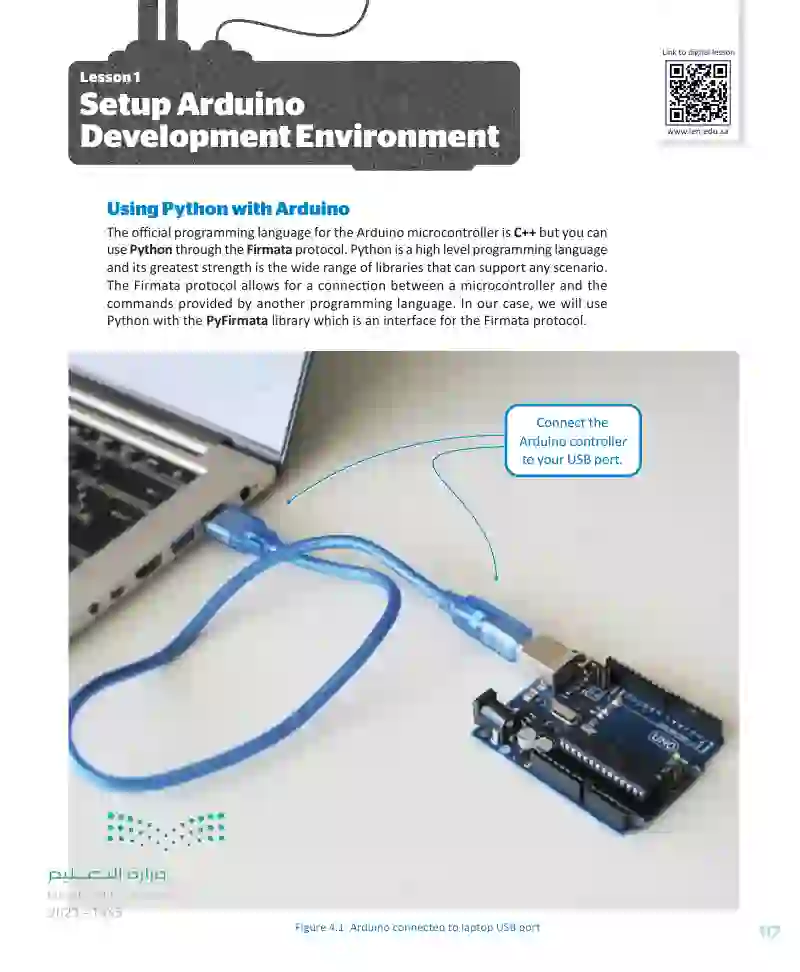
Using Python with Arduino
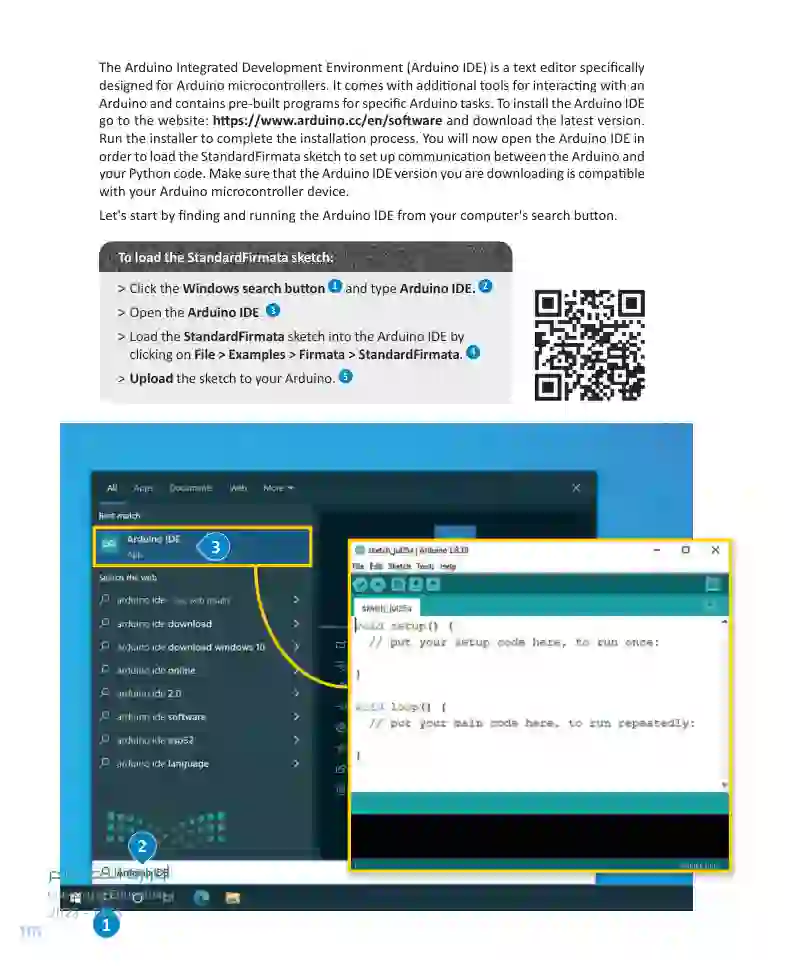
The Arduino Integrated Development Environment (Arduino IDE)
Loading the StandardFirmata sketch:
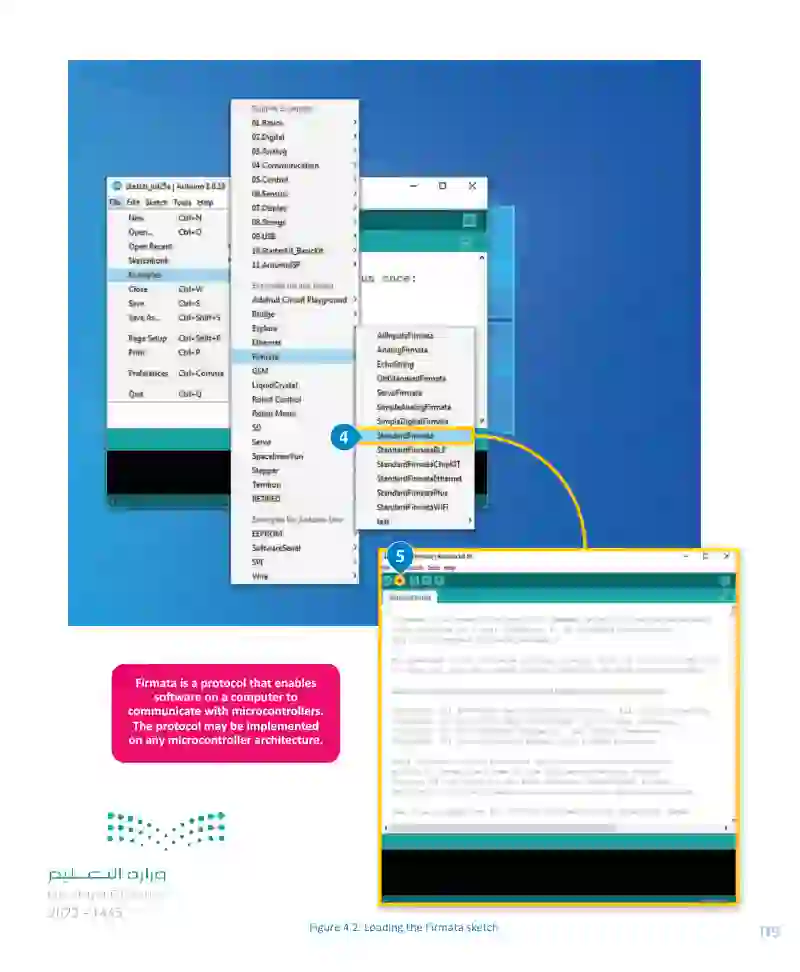
Firmata is a protocol that enables so�ware on a computer to communicate with microcontrollers.
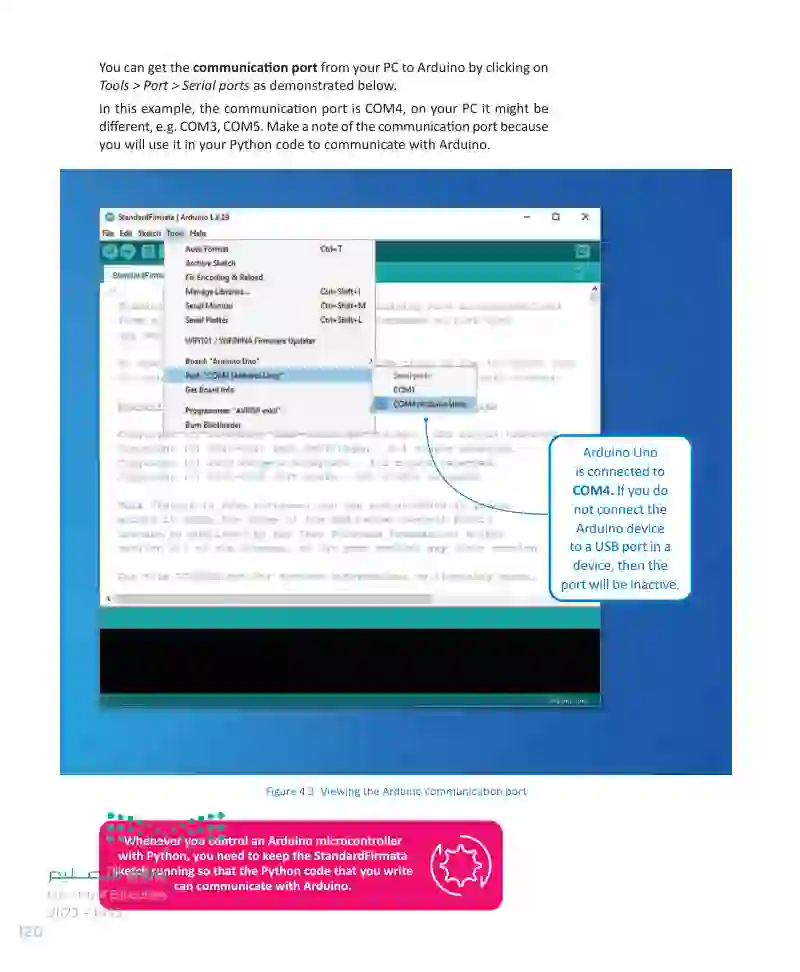
You can get the communication port from your PC to Arduino by clicking on Tools > Port > Serial ports as demonstrated below.
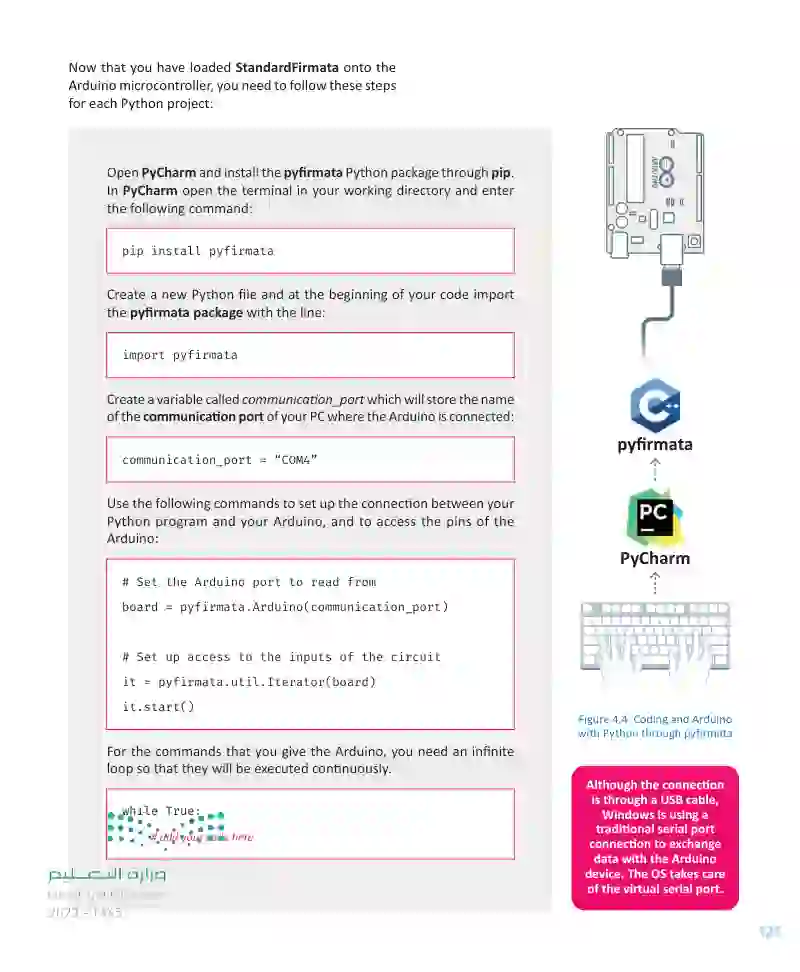
Now that you have loaded StandardFirmata onto the Arduino microcontroller, you need to follow these steps for each Python project:
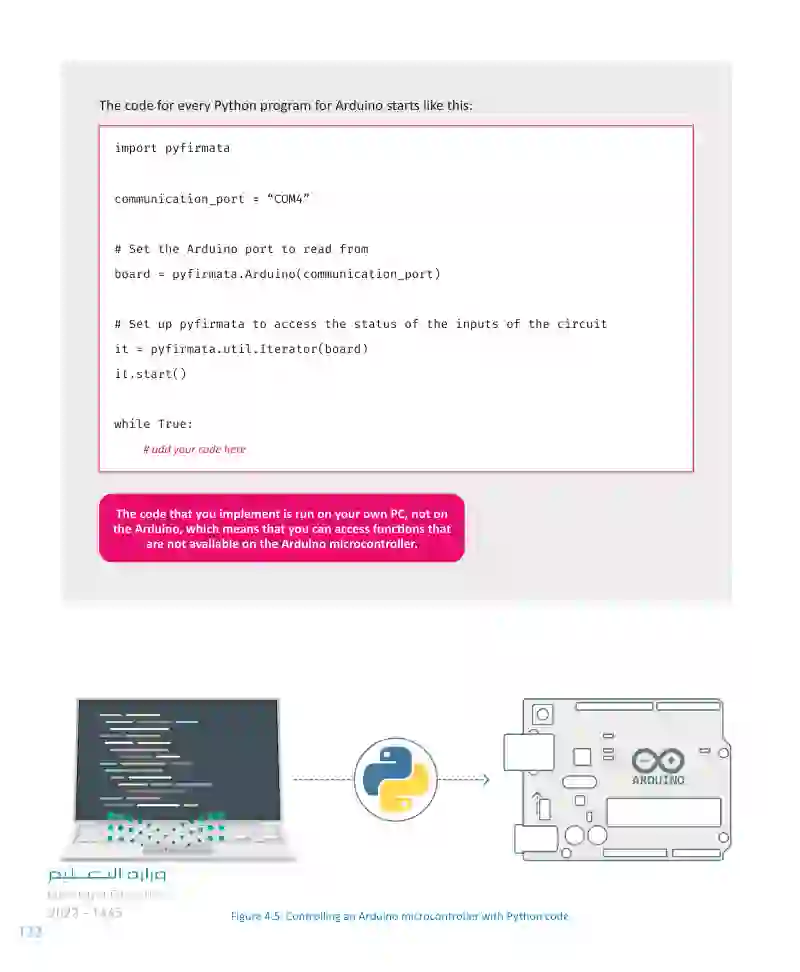
The code for every Python program for Arduino starts like this:
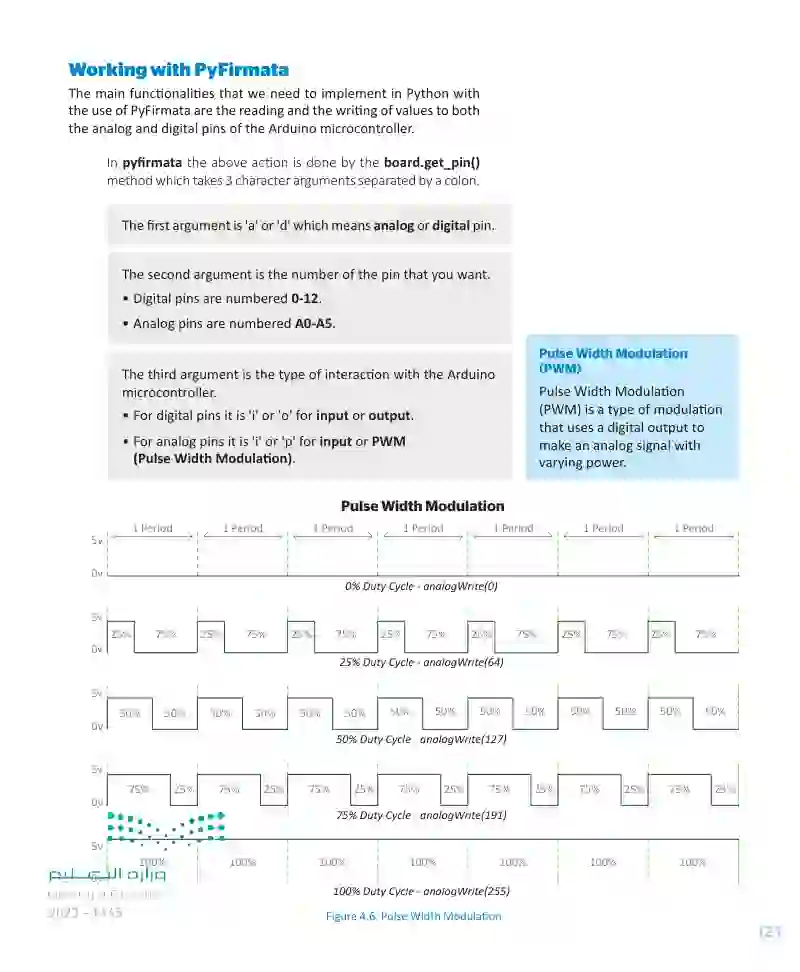
Working with PyFirmata
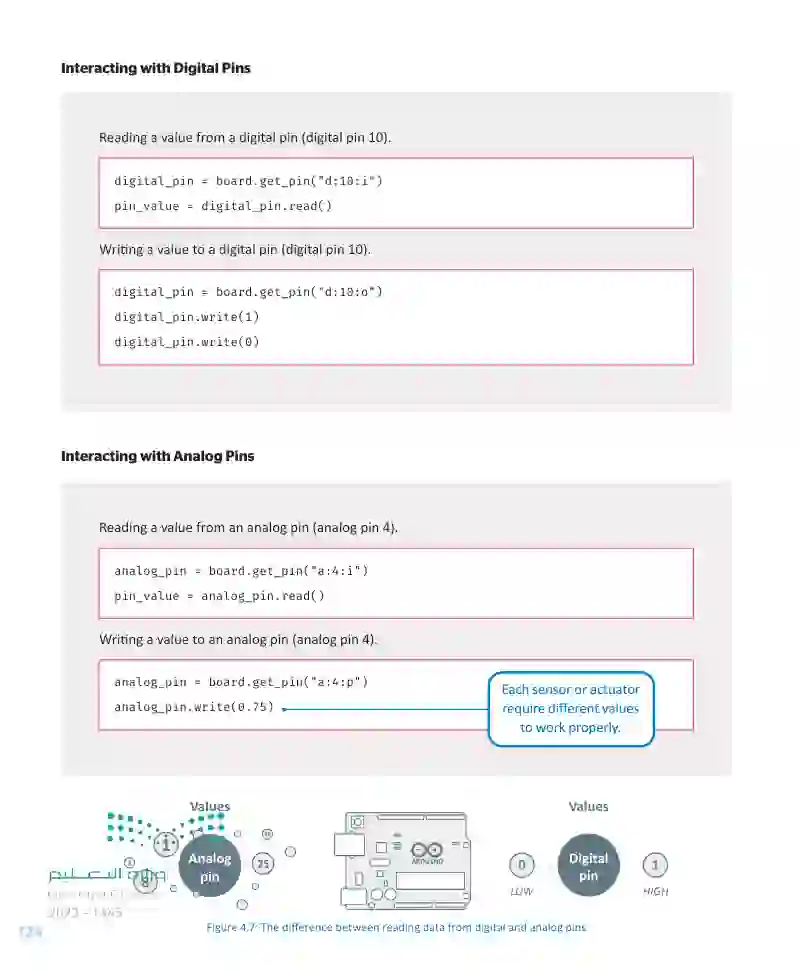
Interacting with Digital Pins
Interacting with Analog Pins
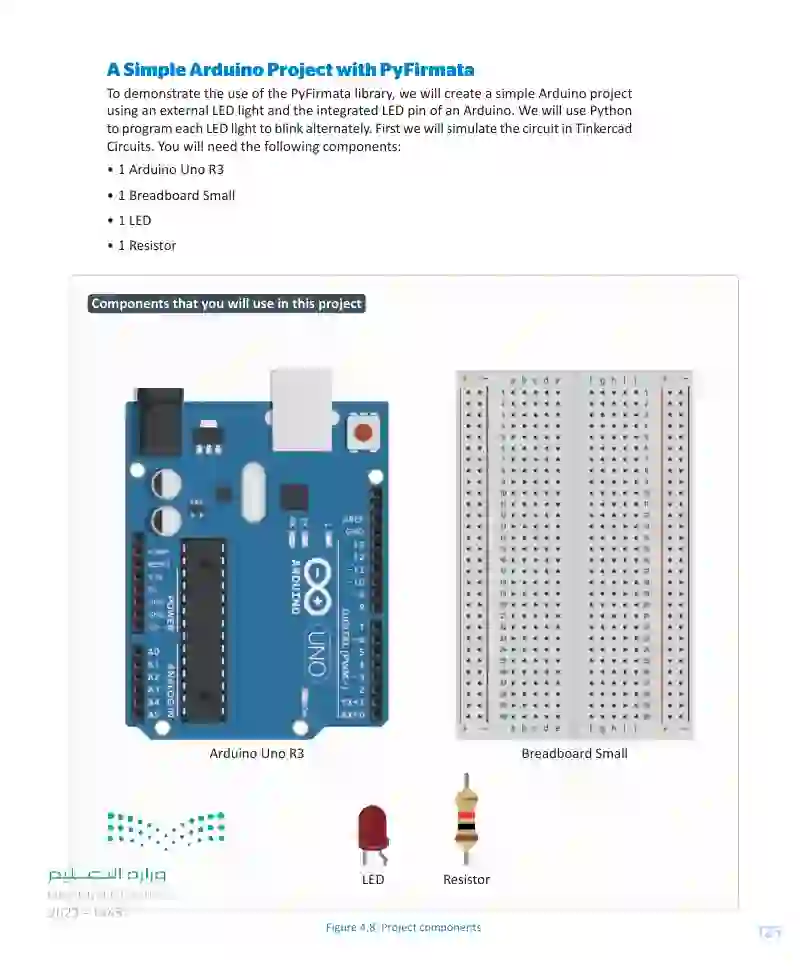
A Simple Arduino Project with PyFirmata
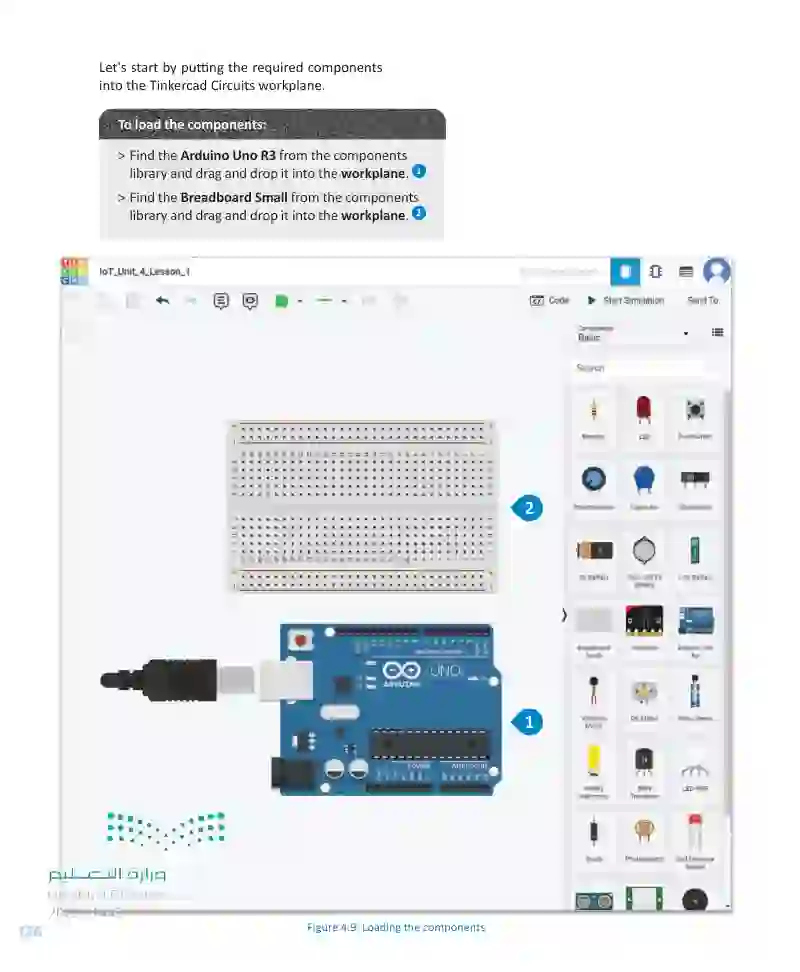
Let's start by putting the required components into the Tinkercad Circuits workplane.
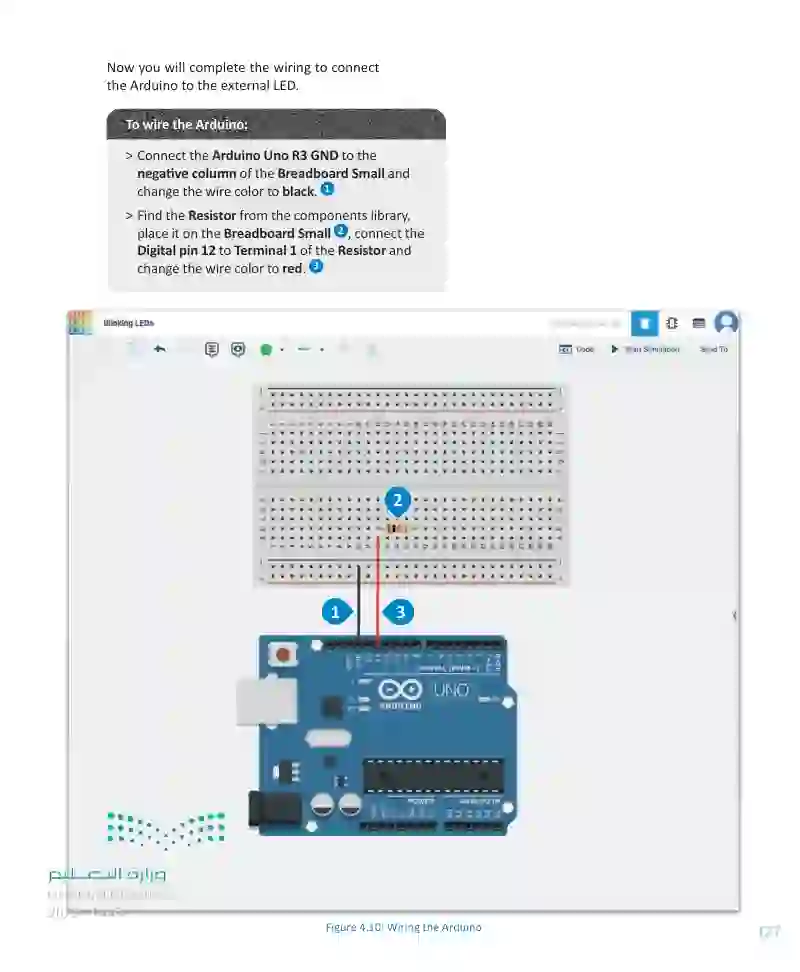
Now you will complete the wiring to connect the Arduino to the external LED.
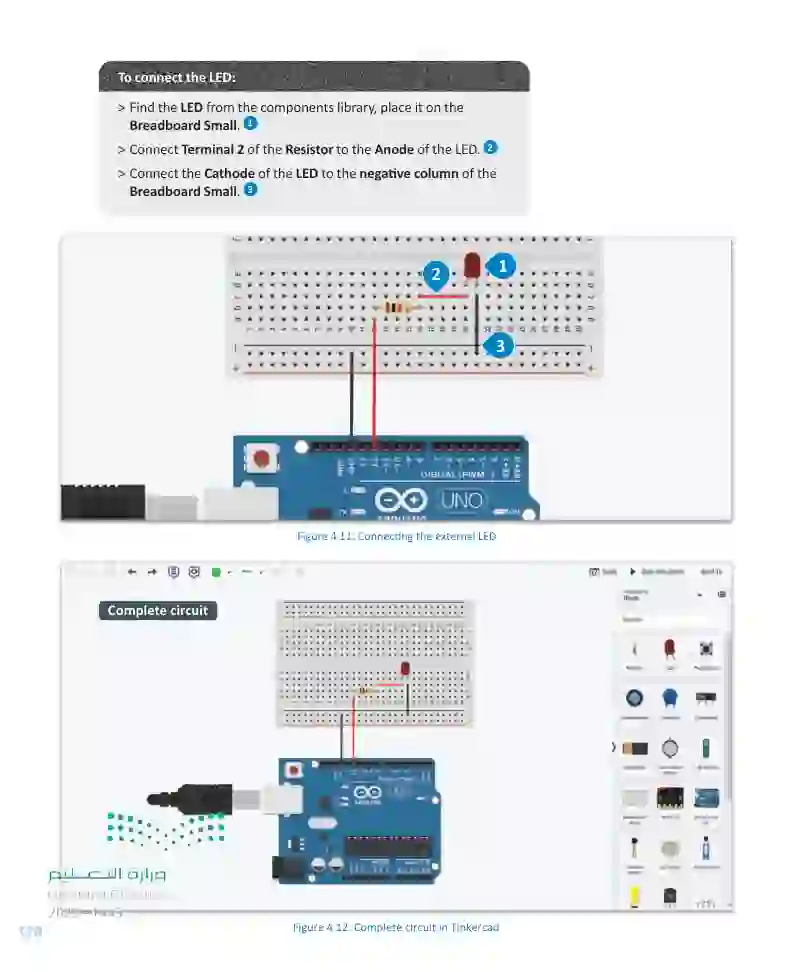
Connecti ng the LED:
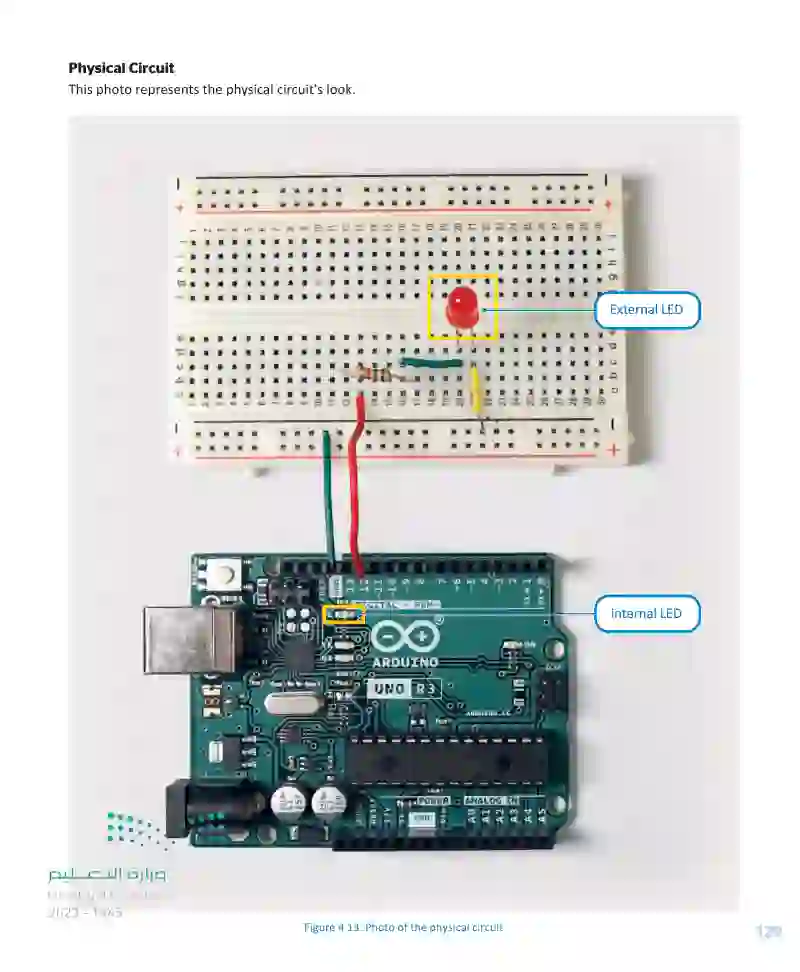
Physical Circuit
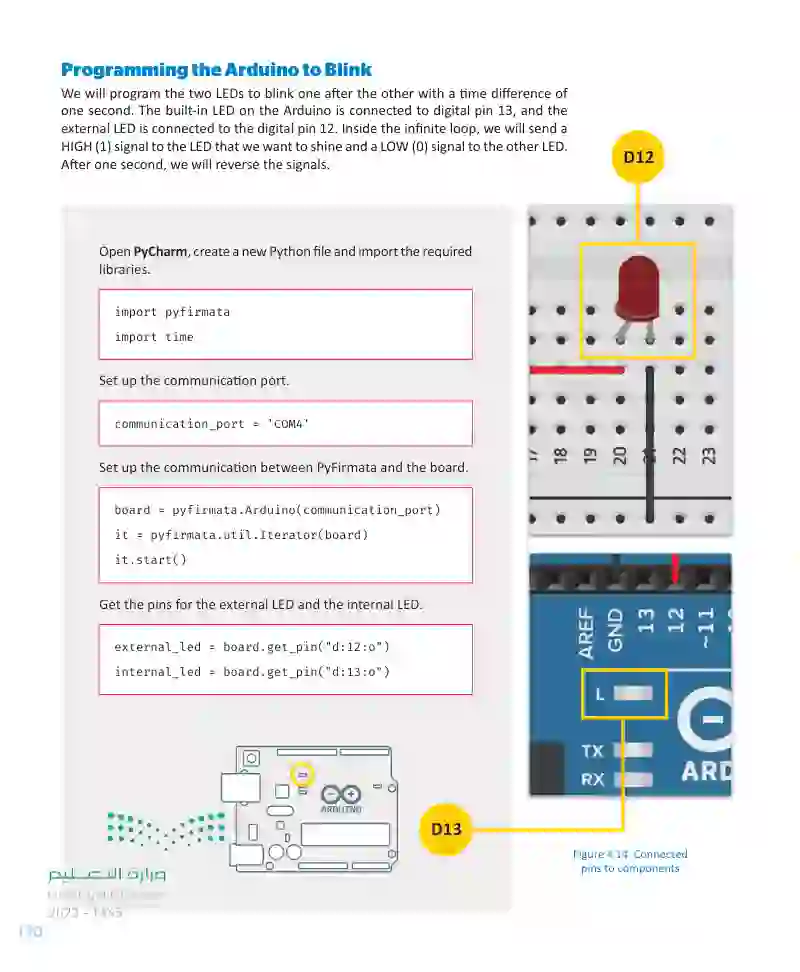
Programming the Arduino to Blink
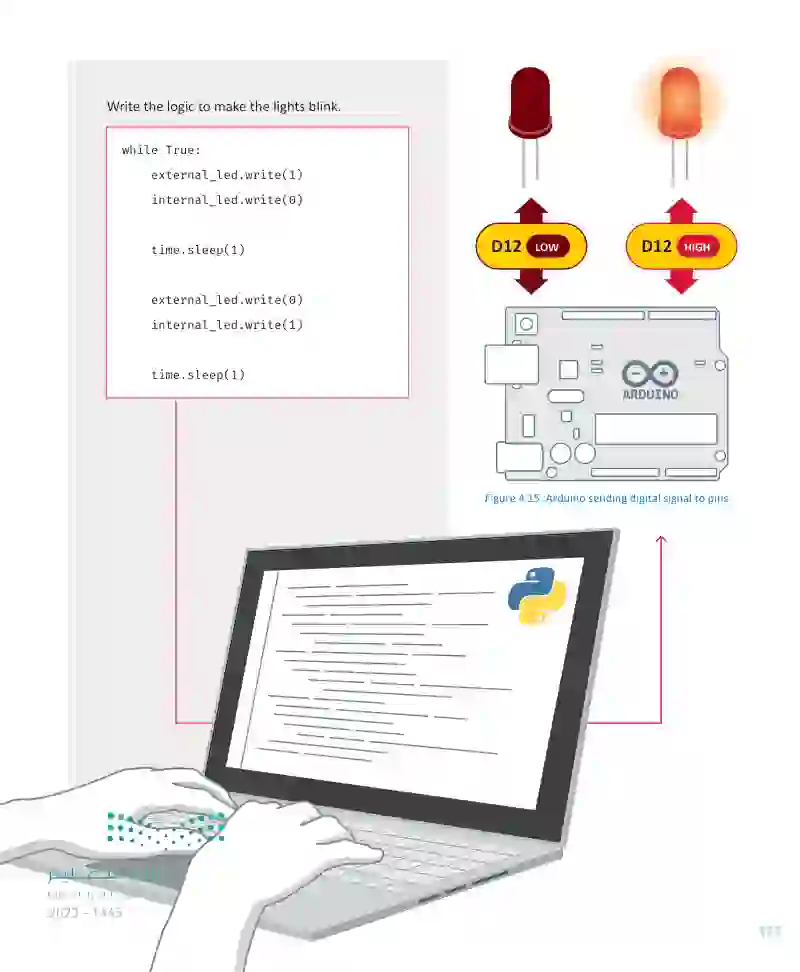
Write the logic to make the lights blink.
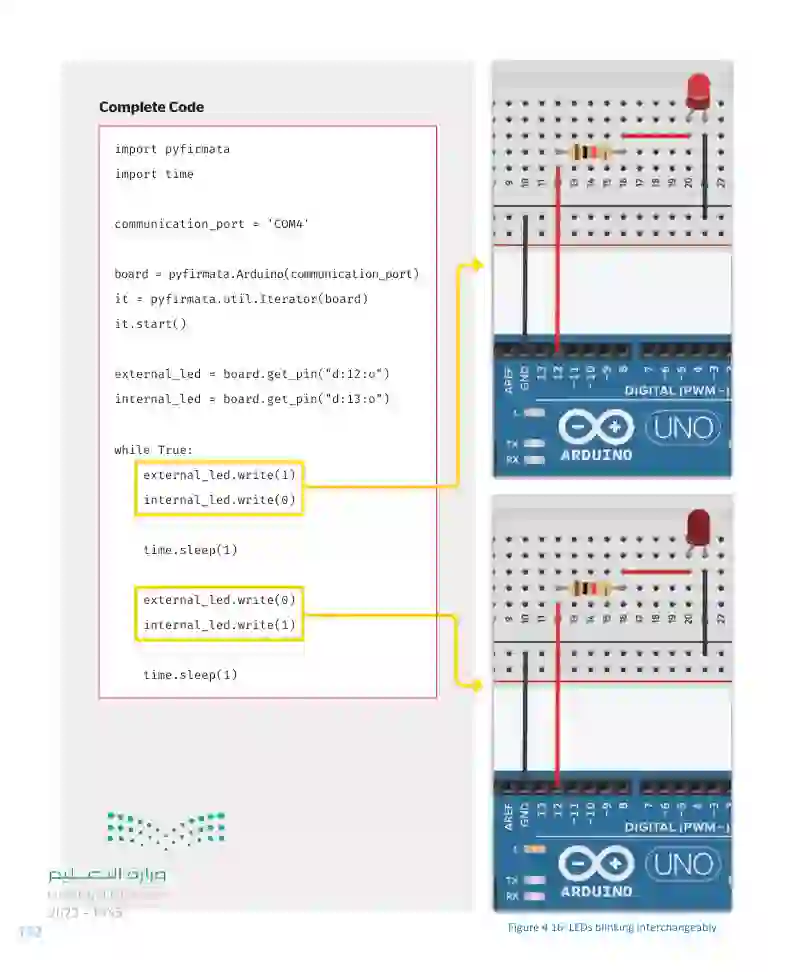
Complete Code
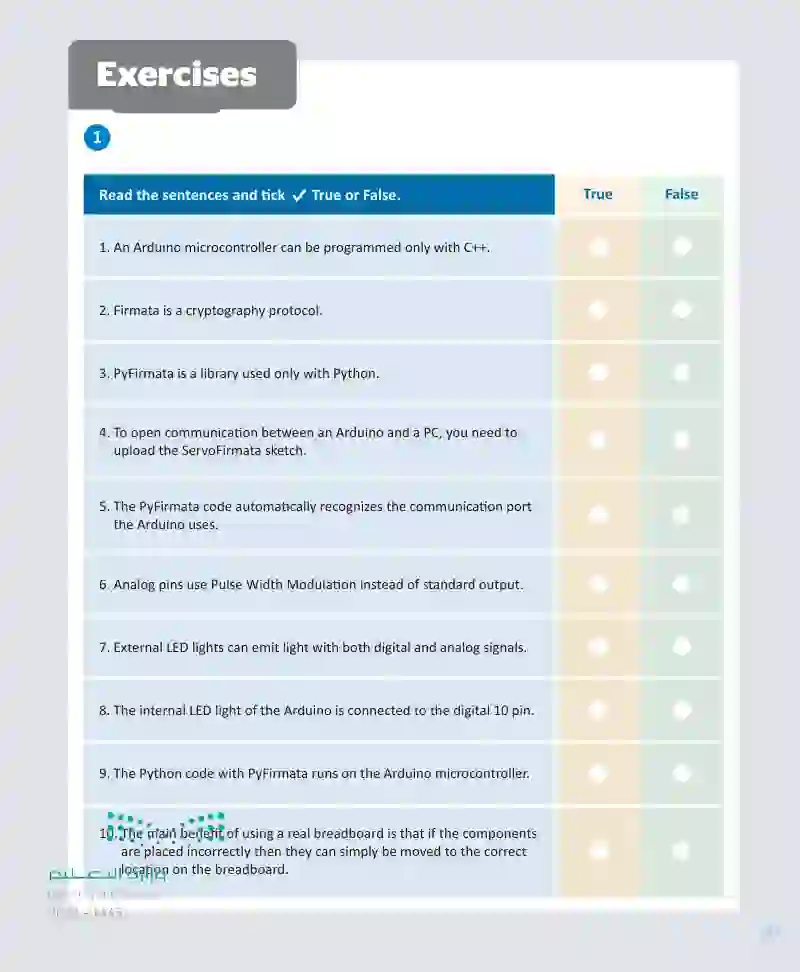
Read the sentences and tick True or False.
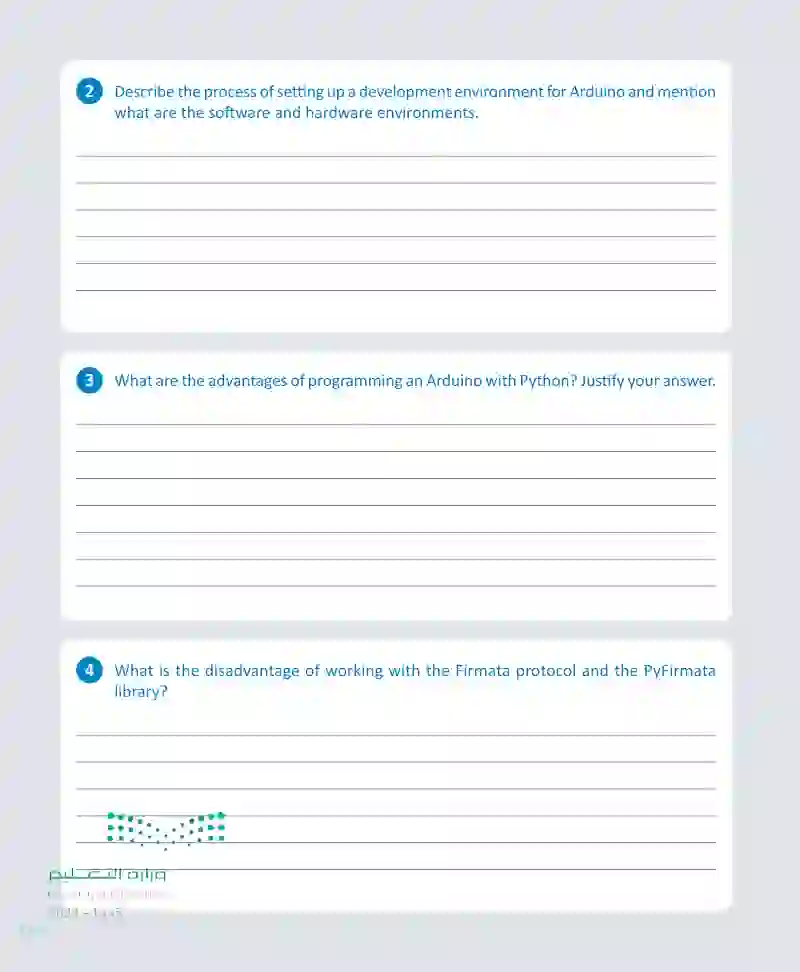
Describe the process of setting up a development environment for Arduino and mention what are the software and hardware environments.
What are the advantages of programming an Arduino with Python? Justify your answer.
What is the disadvantage of working with the Firmata protocol and the PyFirmata library?
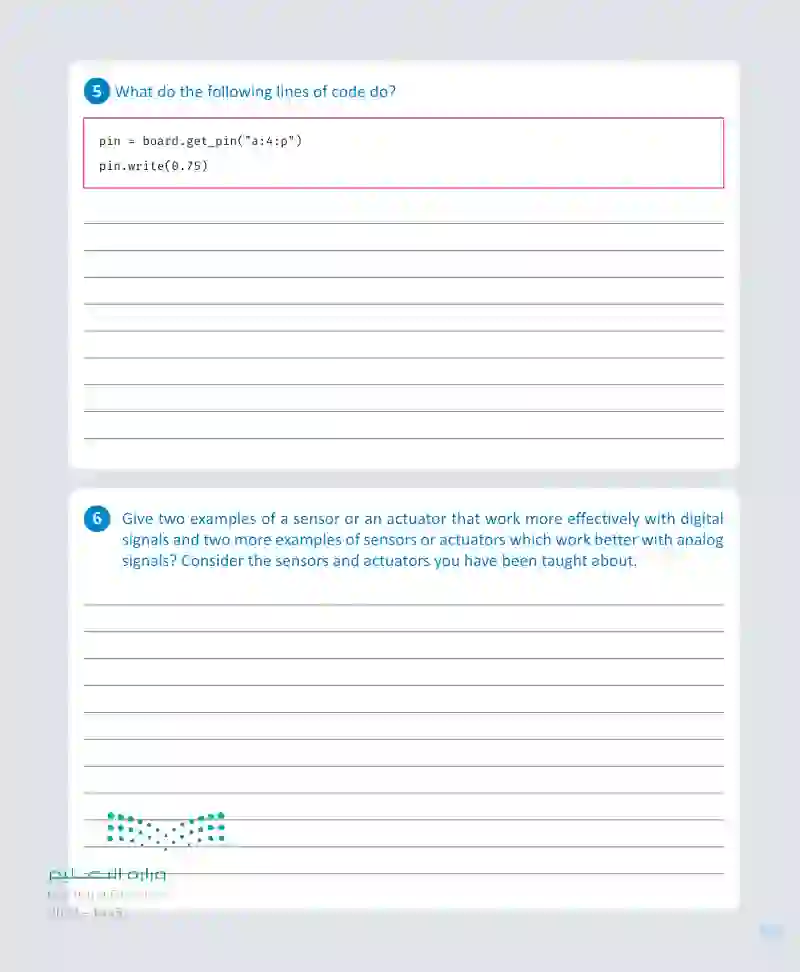
What do the following lines of code do?
Give two examples of a sensor or an actuator that work more effectively with digital signals and two more examples of sensors or actuators which work better with analog signals? Consider the

